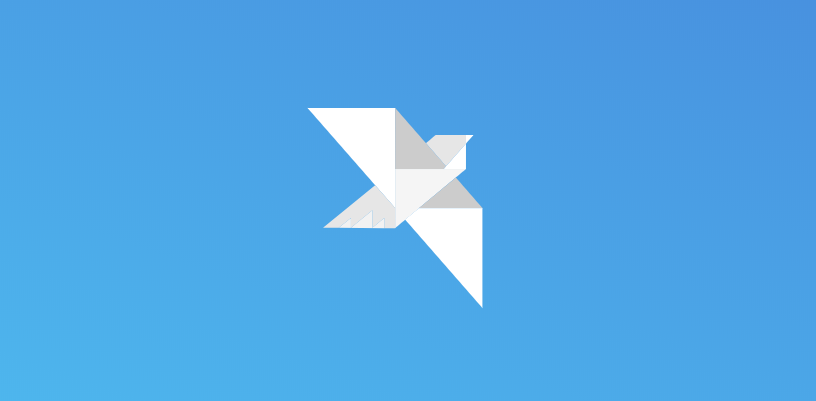
Today is a great day. Today we introduce a new Java microservices frameworks and a new member of MicroProfile family. Please welcome Project Helidon — a new Java open source microservices framework from Oracle.
Helidon is a Greek word for “swallow”, a type of bird that according to Wikipedia has “a slender, streamlined body and long pointed wings, which allow great manoeuvrability and… very efficient flight”. Perfect for darting through the clouds.
Overview
The work on what would become the Helidon Project started some time ago. When we entered the cloud world, and microservices architecture started to become very popular for creating cloud services, we realized that the development experience also needed to change. It’s possible to build microservices using Java EE, but it’s better to have a framework designed from the ground up for building microservices. We wanted to create a lightweight set of libraries that didn’t require an application server and could be used in Java SE applications. These libraries could be used separately from each other, but when used together would provide everything a developer needs to create a microservice: configuration, security, and a web server.
There are already some efforts to build standard microservices frameworks from MicroProfile. MicroProfile is quite popular in the Java EE/Jakarta EE community and provide a development experience similar to Java EE. We like the idea and support the initiative. Helidon implements MicroProfile 1.1. We will continue working on implementing new versions of MicroProfile and intend to support relevant Jakarta EE standards in this area as they are defined.
Helidon is made by Oracle, so don’t be surprised that there will be some useful Oracle Cloud integrations. These are not included in the initial version, but will be added later. Helidon is already used by many internal Oracle projects and these integrations simplify our developers’ lives big time. We believe that will simplify your life as well if you use Oracle Cloud. If not, these integrations are optional.
Landscape
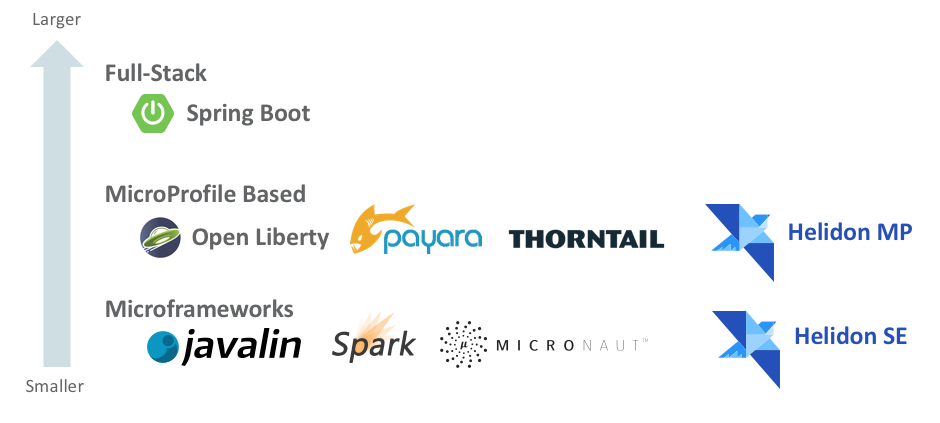
Java Frameworks for writing microservices fall into a few categories. From smaller to larger:
- Microframeworks
Simple, fun, intentionally small feature set. Examples are Spark, Javalin, Micronaut, etc. - MicroProfile
Friendly to Java EE developers but a bit heavier. Some of them are build on top of fully featured Java EE application servers. Examples are Thorntail (was Wildfly Swarm), OpenLiberty, Payara. - Full Stack
Fuller features set such as Spring Boot.
Helidon comes in two flavours and covers two categories — Microframeworks and MicroProfile. It’s up to a developer to choose what to use in their applications.
- Helidon SE — simple, functional, lightweight microframework developed in a modern reactive way. There is no injection “magic”. No special runtime required. JDK is used as runtime.
- Helidon MP — Eclipse Microprofile implementation providing development experience familiar to Java EE/Jakarta EE developers.
Architecture
Helidon’s high level architecture is shown on the picture below.
- Helidon SE components are marked green. These are Config, Security and RxServer.
- The Java EE/Jakarta EE components we use are marked grey. These are JSON-P, JAX-RS/Jersey and CDI. These components are required for MicroProfile implementation.
- Helidon MP is a thin layer on top of Helidon SE components.
- Oracle Cloud services components are marked red and used in both Helidon SE and Helidon MP.
Usage and Samples
Setup
The easiest way to start using Helidon is if you use a Maven project with Java 8 (or higher version) and configure the Helidon bom-pom:
Helidon SE
Helidon SE is the base to build lightweight reactive microservices.
A Hello world implementation:
This would start webserver on a random (free) port and expose endpoint /hello.
Imports:
Dependency needed to start this example (version not required if you used bom-pom):
Adding metrics to SE
We have provided an implementation of MicroProfile Metrics interfaces also for Helidon SE (without support for injection, as that is not included in SE).
Updated source code with metrics:
This requires a new dependency:
New endpoints are available:
- /metrics
All metrics (base, vendor and application) - /metrics/application/helloCounter
The metric created in our hello world application
Helidon MP
Helidon MP is Eclipse Microprofile implementation and a runtime for microservices.
A Hello world implementation that uses metrics to meter the invocations.
You must create a JAX-RS resource class to process your requests:
And then start the server with this resource:
You also need to create beans.xml file in the src/main/resources/META-INF directory to trigger CDI injection in this project:
This would start a webserver on the default port (7001) and expose endpoint /hello.
The following endpoints are available:
- http://localhost:7001/hello
The hello world example - http://localhost:7001/metrics
MicroProfile Metrics endpoint - http://localhost:7001/metrics/application/com.oracle.tlanger.HelloWorld.hello
The metric of our endpoint - http://localhost:7001/health
MicroProfile Health endpoint
Plans
We have so many plans, I could write a separate article about them.
Our short term goal is to advertise Helidon in the Java community. We are planning to talk about Helidon at some conferences. We have four Helidon-related Oracle Code One 2018 talks planned. We have also submitted talks to EclipseCon Europe 2018 and will participate in the Jakarta EE/MicroProfile Community Day there. Some learning materials such as screen casts and YouTube videos are also on the way.
From the technical perspective, we are working on implementing the next MicroProfile versions very soon. We are also working on Oracle GraalVM support, which looks like a great feature for Helidon SE users.
We are working on some other cool stuff and features, but I can’t show all my cards now. Stay tuned, we will announce new features as soon as the are ready.
Useful Links
- Helidon web site: http://helidon.io
- Helidon on Twitter: @helidon_project
- GitHub repository: https://github.com/oracle/helidon
You may find it interesting
- The original internal name of the project was J4C (Java for Cloud).
- The Helidon team consists of two smaller teams in Prague (Czech Republic) and in the U.S.
- Helidon is used in more than 10 projects within Oracle.
- Some Helidon components are still in development and not open sourced yet.
Original article on my Medium blog.
Congratulations!
I’ll just give a presentation in a couple of weeks on microprofile.
Now I will add Helidon to the presentation 🙂
LikeLike
It would be great! 👍
LikeLiked by 1 person
[…] the different servers from Dmitry Kornilov shown below and the associated article can be seen here (who also happens to the Lead Engineer for […]
LikeLike